CTF Tools
Networking
The ctf.network.tcp package provides three important classes to you: Server, Connection and TestConnection. The first is used for establishing TCP/IP servers (e.g. a CTF simulator), the second is used for connecting to TCP/IP servers (e.g. like a CTF controller would like to do), and third is used during testing.
Server
The ctf.network.tcp::Server class is used to host TCP/IP servers (Transmission Control Protocol / Internet Protocol - the same protocol that HTTP, FTP, POP3, etc. run on top of). It is a very simple class.
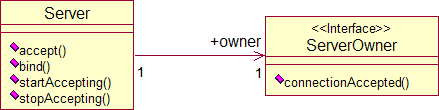
When instantiating a server, you must first bind to a TCP port (which should be greater than 1024 unless you're implementing a known Internet service). If the port is not in use, bind() succeeds, and you may start accepting connections:
Calling startAccepting(int) will start a new thread, which accepts connections until the specified number of connections have been accepted. New connections are passed to Server's "owner" through the connectionAccepted() method. The ctf.network.tcp::Connection is a TCP connection to the client, which is how communication with the client actually occurs (see below).
Calling accept() directly will accept one connection synchronously (i.e. the call will block until a client connects).
Connection
Communication over a TCP/IP connection is accomplished with the ctf.network.tcp::Connection class.
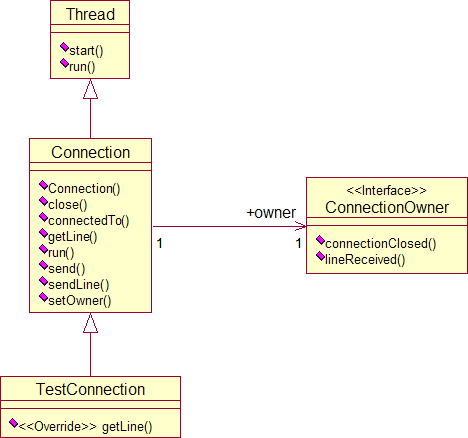
Connecting to a TCP/IP server is very simple, as is sending and receiving text:
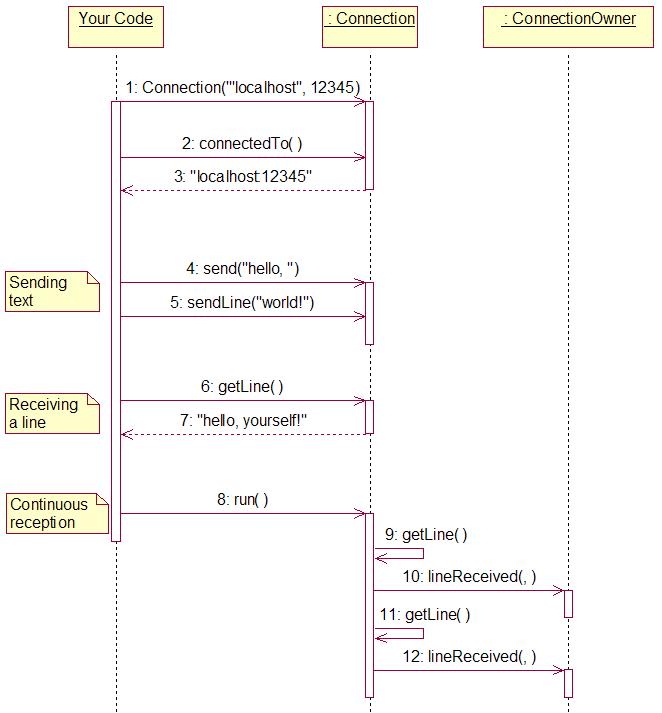
Calling run() will make the Connection enter a loop of
reflexive getLine() calls, which it will only exit if there is a
communication problem (e.g. the other side of the connection is
closed).
Since Connection extends Thread, you may also call start(), which will
start a new thread to run() in the background.